728x90
반응형
기존에 만들었던 영화목록은 따로 파라메터를 받지 않고 그냥 url뒤에 필요한 값들을 하드코딩해서 보냈었다.
이번에는 검색어와, 가져 올 영화갯수, 페이지 처리를 해보겠다.
근데 우선 알아야 할 것은 해당 데이터가 데이터베이스에 있는 것이 아니라, API로 그때그때 가져오는 거라,
기존의 페이지네이션 처리 방식이 다르다.
나는 그냥 페이징 계산 안하고 [이전], [다음] 버튼 정도만 구현하였다.. 간단히 코드랑 결과화면만 찍겠다.
MovieList.vue <template>
<javascript />
<template>
<div>
<section class="py-5 text-center container">
<div class="row py-lg-5">
<div class="col-lg-6 col-md-8 mx-auto">
<h1 class="fw-light">Album example</h1>
<p class="lead text-muted">Something short and leading about the collection below—its contents, the creator, etc. Make it short and sweet, but not too short so folks don’t simply skip over it entirely.</p>
<p>
<a href="#" class="btn btn-primary my-2">Main call to action</a>
<a href="#" class="btn btn-secondary my-2">Secondary action</a>
</p>
</div>
</div>
</section>
<!-- 검색버튼 -->
<div class="input-group mb-3">
<!-- v-model="query"로 주어 양방향 바인딩 걸어줌-->
<input type="text" class="form-control" placeholder="Recipient's username" aria-label="Recipient's username" aria-describedby="button-addon2" v-model="query">
<!-- 버튼 클릭시 searchMovie 함수 호출, 인자 12는 영화의 1~12까지 가져온다는 의미 -->
<button class="btn btn-outline-secondary" type="button" id="button-addon2" @click="searchMovie(12)">Button</button>
</div>
<!-- 실제 영화 목록 -->
<div class="album py-5 bg-light">
<div class="container">
<div class="row row-cols-1 row-cols-sm-2 row-cols-md-3 g-3">
<!-- 가져온 영화 목록이 있을 때 보여줌 ( v-show 디렉티브 )-->
<div v-show="movieList.length!=0" class="col" v-for="data in movieList" :key="data">
<div class="card shadow-sm">
<img class="imageStyle" :src="data.image" style="width:100%; height:300px;">
<div class="card-body">
<p class="card-text">{{data.title}}</p>
<p class="card-text"></p>
<div class="d-flex justify-content-between align-items-center">
<div class="btn-group">
<button type="button" class="btn btn-sm btn-outline-secondary">상세보기</button>
</div>
<small class="text-muted">{{data.regDate}}</small>
</div>
</div>
</div>
</div>
<!-- 가져온 영화 목록이 없을 때 보여줌 ( v-show 디렉티브 ) -->
<div v-show="movieList.length==0" class="noneMovie">
영화 제목을 입력하여 주십시오.
</div>
</div>
</div>
</div>
<!-- 페이징 추가 -->
<nav id="pagination" aria-label="...">
<ul class="pagination">
<li class="page-item">
<!-- count 함수 호출, 인자로 'decrement' 줌 -->
<a class="page-link" href="#" @click="count('decrement')">이전</a>
</li>
<li class="page-item" aria-current="page">
<!-- 현재페이지 표출 -->
<a class="page-link" href="#">{{Math.ceil(start/12)}}</a>
</li>
<li class="page-item">
<!-- count 함수 호출, 인자로 'increment' 줌 -->
<a class="page-link" href="#" @click="count('increment')">다음</a>
</li>
</ul>
</nav>
</div>
</template>
점점 코드가 길어지니 보기 싫어지네..
검색버튼, 페이징을 추가했다. 관련 함수는 아래
MovieList.vue <script>
<javascript />
<script>
export default ({
data () {
return {
movieList : [],
query : '-', //검색어
display : 12, // 한번에 가져 올 영화갯수
start : 1, // 몇번째부터 몇번째까지 가져올 지 정함 (페이징 개념)
}
},
mounted () {
this.getMovieList(); // MovieList가 화면에 랜더링 되었을 때 getMovieList 함수를 호출한다.
},
methods : {
getMovieList : function(){ // API요청을 통해 영화목록을 받아온다.구보씨 일보
//검색어가 없을 시 - 를 보냄 (네이버 오픈API의 query는 필수값이며, default가 - 이기 때문)
this.query = '-';
this.searchMovie(12);
},
searchMovie : function(start){
this.movieList = [];
this.start = start;
const URL = "/v1/search/movie.json"; /*URL*/
//기존 URL에 파라메터들을 동적으로 받는 cURL 선언
const cURL = URL + '?query=' + this.query + '&display=' + this.display + '&start=' + this.start;
const clientId = 'n9KqdakFPww8Um1PXnUK';
const clientSecret = '8Eq-----S3';
this.$axios.get(cURL, //cURL로 API요청
{headers : {
'Accept' : 'application/json',
'X-Naver-Client-Id': clientId,
'X-Naver-Client-Secret': clientSecret,
}
}).then((response) => { // 실제 API를 요청한다/
this.movieList = this.movieList.concat(response.data.items);
})
},
count : function(param){
if(param=='increment'){
this.start += 12;
}else{
if(this.start<=12){
alert("최소값은 1페이지 입니다.");
return false;
}
this.start -= 12;
}
this.searchMovie(this.start);
},
}
})
</script>
MovieList.vue <style>
<javascript />
<style scoped>
.mb-3 {width: 600px; position: relative; left: 1950px}
.noneMovie {position: relative;left: 50%;}
#pagination {position: relative;left: 50%; margin-top: 70px;}
</style>
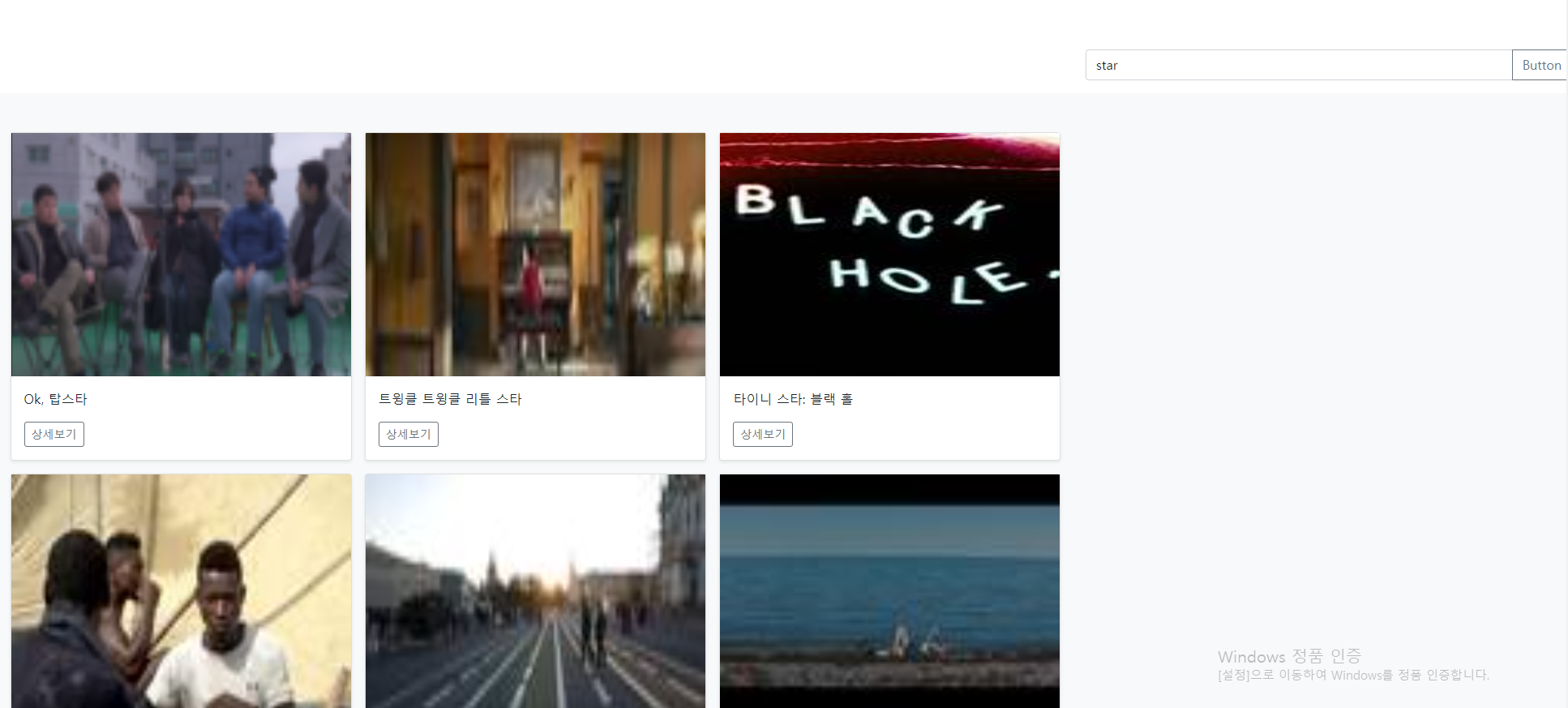
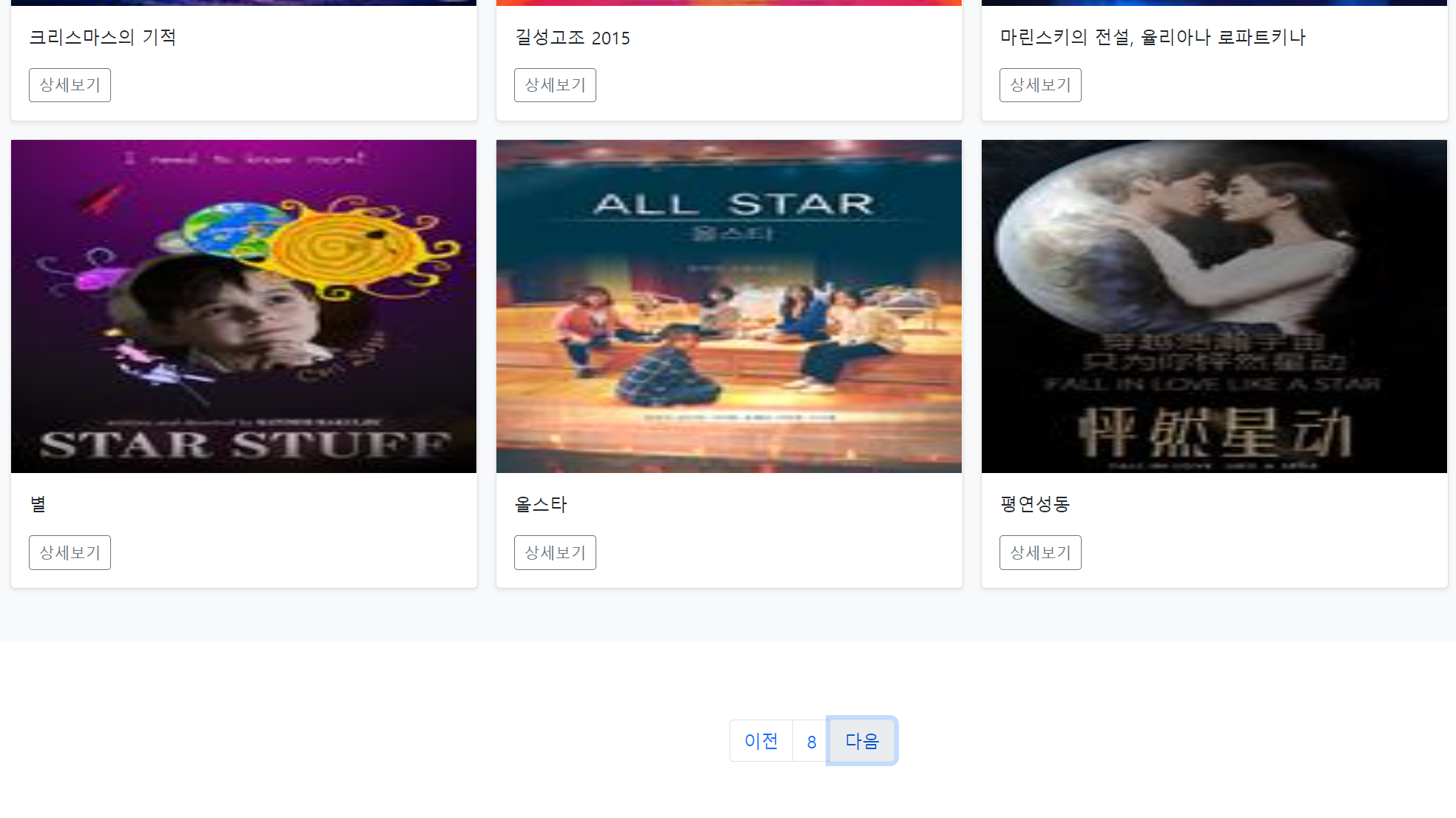
검색과 페이지 처리까지 잘 된다. 물론 수정할 게 많은 코드인데 API로 가져오는 한계라면 한계인 듯 하다.
다음에는 페이지 이동을 한번 해봐야겠다. 그럼 Router 공부를 해야...
728x90
반응형